Codux Help Center
Browse our articles to find the answers you need
Working With Any External Component Library
Codux detects third party components using a JSON file that you'll include in the project's root directory, in which there are mappings between components and their import paths. For example, to use the Ant Design component library, you'd create the file, named
codux-external-components.json
, like this:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
[ { "name": "Ant Design", "components": [ { "filePath": "antd", "exportName": "Progress", "name": "Progress Bar" }, { "filePath": "antd", "exportName": "Button", "name": "Button" } ] }
Codux uses the information provided in the JSON file to present components from your dependencies in the Add Elements panel. Users will see each of the components exactly as they were described in the JSON file. For example, with the JSON from the configuration example above, the Add Elements panel shows the Ant Design library like this:
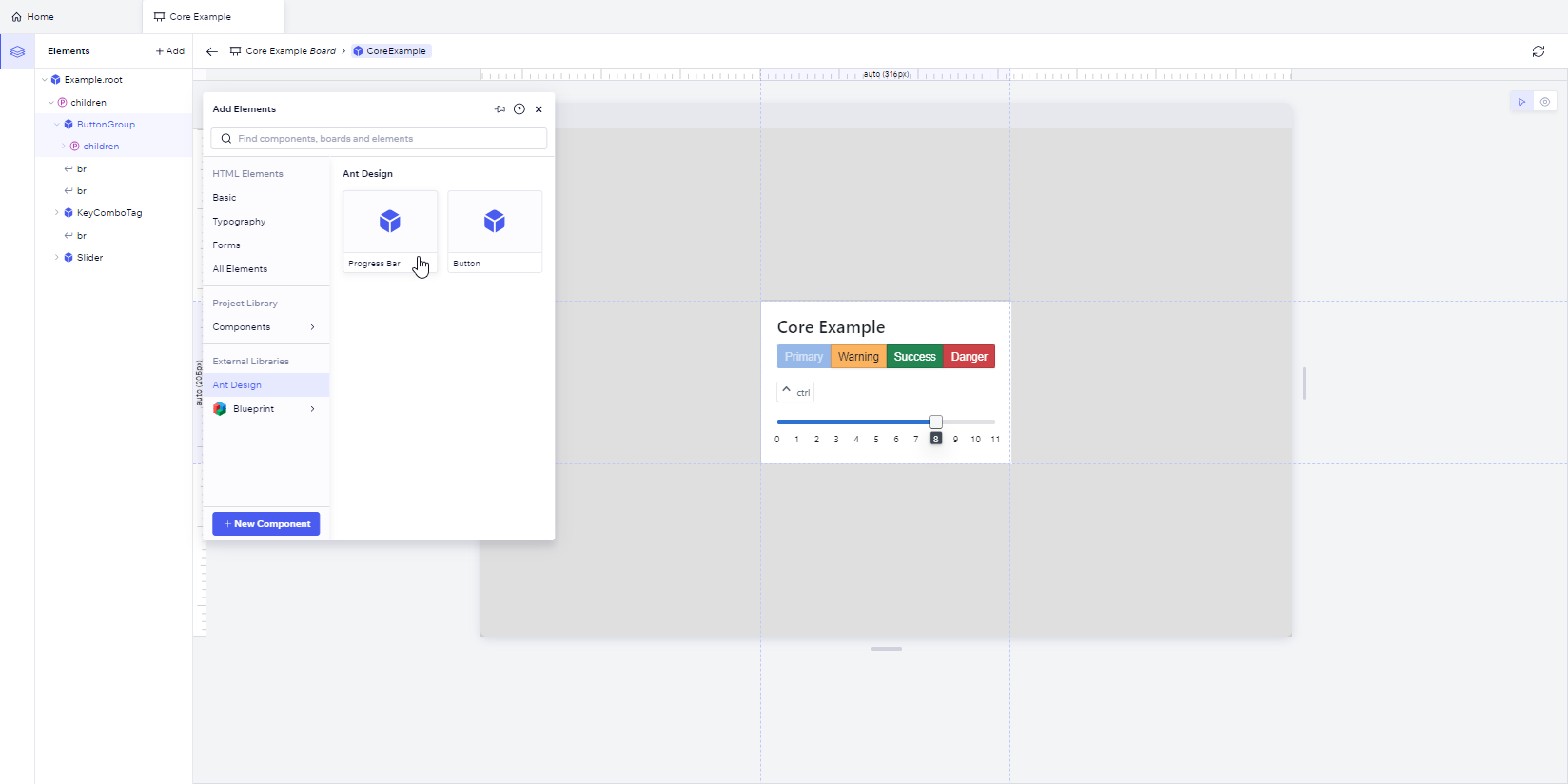
Tip!
Regex can be very useful for parsing and manipulating files. To automate the process of adding new components to the file, you could use Regex to search for the correct insertion point in the file and then insert the new component's details in the correct format.
Configuration
Key | Type | Description |
---|---|---|
name | string | Display name for library in the Add Elements panel. |
components | Array<ComponentConfig> | Array of components from your dependencies.
|
ComponentConfig
Key | Type | Description |
---|---|---|
filePath | string | Absolute path to file. |
exportName | string | Either the export name, or "default". |
name | string | Display name for component in Add Elements panel. |
Note:
This is an experimental feature and may undergo changes to its implementation in the future.
Note:
Codux does not validate library names, the existence of components in the project, or component names or paths. Components as listed in the Add Elements panel exactly as they are in the JSON file. Users will get an error message as soon as they add a component to the Elements panel that wasn't configured correctly in the file.
Note:
Components added via the Add Elements panel contain no property values. This means that the user will need to go to the Properties panel to add values after adding the component. In cases where the component has required props, Safe Render will kick in to indicate this, and render the rest of the board around the temporarily-missing propless component as good as possible.
For information on adding cover images so that you can see what the third party components look like in the Add Elements panel before adding them to a target, see here.
Named vs Default Exports
When you include a third party component in your JSON file and then add that component to the Elements panel, Codux adds the import statement to the project's code automatically. To do this correctly, Codux needs to know if it is a named or a default export.
In general, when you import a named component in TypeScript React, the import statement refers to the component by the name used in the export. For example:
1 2 3 4 5 6 7
// export export const Lollipop = () => { ... } // import import { Lollipop } from "./sweet-components/lollipop"
When you use a default export, on the other hand, you can give it whatever name you want (without using curly brackets). For example:
1 2 3 4 5
// export export default Lollipop // import import Candy from "./sweet-components/lollipop"
There are different reason why a developer will choose to use one export technique over another. Regardless of the technique used, however, you'll want to configure the JSON file according to the library's export. Set the
exportName
field of the component in the JSON file with a default export as "default", or give the exportName
field a name of your choosing if it uses a named export. Here is a JSON configuration for a default export:
1 2 3 4 5 6
{ "comments": "A default export in codux-external-components.json", "filePath": "sweet-components/lollipop", "exportName": "default", "name": "Lollipop" }
Here is a JSON configuration for a named export:
1 2 3 4 5 6
{ "comments": "A named export in codux-external-components.json", "filePath": "react-color", "exportName": "SketchPicker", "name": "SketchPicker" }
Was this article helpful?